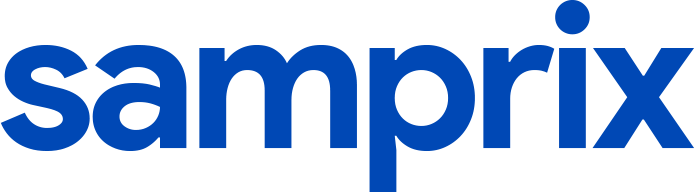
Optimize your audio with our free online Audio Processor. Increase volume, reduce noise, and enhance sound clarity with ease.
Compress your images online for free without compromising quality. Reduce image file size for faster websites and better performance.